In the ever-evolving world of Flutter app development, it’s crucial to stay up-to-date with the best practices that can simplify the process and make your development workflow more efficient. To help you achieve that, we have compiled a comprehensive list of the 12 best practices to simplify Flutter app development in 2023.
12 Best Practices to Simplify Flutter App Development
These practices are carefully curated to address various aspects of Flutter development, ranging from code organisation to UI design and testing. By incorporating these practices into your development routine, you can optimize your codebase, improve performance, and ensure a smooth user experience.
Throughout this guide, we will cover essential practices such as making the build function pure, using operators smartly, leveraging streams judiciously, and utilising raw strings. We will also explore the advantages of relative imports, using SizedBox instead of Container, and logging instead of print statements.
Additionally, we will delve into significant practices like avoiding explicit initialisation of variables as null, embracing the const keyword whenever possible, and employing robust state management solutions. We will also emphasise the importance of adhering to Material Design guidelines and writing automated tests for ensuring code quality and reliability.
By following these 12 best practices, you will simplify your Flutter app development process, improve maintainability, and deliver high-quality applications to your users. Let’s dive in and explore these practices in detail to enhance your Flutter development skills in 2023.
1. Building a Pure and Efficient Build Function in Flutter
1st of 12 Best Practices to Simplify Flutter App Development, In Flutter, the build function is responsible for creating the UI of a widget. It’s good practice to keep the build function pure, meaning it should only be used for UI rendering and not for other computations or side effects. By keeping the build function pure, you ensure that the UI remains consistent and predictable. Here’s an example:
class MyWidget extends StatelessWidget {
final String text;
const MyWidget(this.text);
@override
Widget build(BuildContext context) {
return Text(text);
}
}
2. Reducing Code Length with Smart Operator Usage in Flutter
2nd of 12 Best Practices to Simplify Flutter App Development, When it comes to Flutter app development, optimizing code length and reducing the number of lines can significantly improve readability and maintainability. In this guide, we will explore the smart use of operators to streamline code execution and enhance efficiency. By leveraging techniques such as the cascades operator, spread collections, null safe (??) and null aware (?.) operators, and avoiding the use of the “as” operator, you can write more concise and effective code in Flutter.
Use Cascades Operator: The cascades operator (..) allows you to perform multiple operations on the same object without repeating the object’s name. This helps reduce the number of lines and improves code readability. Here’s an example:
myObject..method1()..method2()..method3();
Use Spread Collections: The spread operator (…) allows you to spread the elements of a collection into another collection. This simplifies the process of combining or cloning collections, reducing code redundancy. Here’s an example:
List<int> list1 = [1, 2, 3];
List<int> list2 = [4, 5, ...list1, 6, 7];
Use Null Safe (??) and Null Aware (?.) Operators: The null safe operator (??) allows you to provide a fallback value when encountering null values, reducing the need for conditional checks. The null aware operator (?.) allows you to access properties and methods without causing a null reference exception. Here’s an example:
String name = nullableName ?? 'Unknown';
int length = object?.property?.length;
Avoid Using “as” Operator: Instead of using the “as” operator for type casting, it’s recommended to use the “is” operator combined with type checks. This helps ensure type safety and avoids potential runtime errors. Here’s an example:
if (myObject is MyClass) {
MyClass myClass = myObject as MyClass;
// Do something with myClass
}
By applying these operator techniques, you can write more concise and efficient code in Flutter, reducing lines of execution while maintaining code readability and reliability.
3. Harnessing the Power of Raw Strings in Flutter
3rd of 12 Best Practices to Simplify Flutter App Development, Raw strings (denoted by an ‘r’ prefix) are useful when dealing with strings that contain escape characters or special characters. They allow you to write the string without escaping those characters. Here’s an example:
String path = r'C:\path\to\file.txt';
print(path); // Output: C:\path\to\file.txt
4. Optimising Imports with Relative Import Paths in Flutter
4th of 12 Best Practices to Simplify Flutter App Development, When importing files in Flutter, it’s generally better to use relative imports instead of absolute imports. Relative imports make it easier to refactor or move files within your project without updating import statements. Here’s an example:
import '../models/user.dart';
import '../services/api.dart';
5. Improving Layout Efficiency with SizedBox in Flutter
5th of 12 Best Practices to Simplify Flutter App Development, If you need to add empty space or define a fixed size widget, it’s more efficient to use the SizedBox widget instead of a Container. SizedBox is a lightweight widget that doesn’t have any decoration or child, while Container comes with additional overhead. Here’s an example:
SizedBox(width: 20, height: 20);
6. Enhancing Debugging with Logging in Flutter
6th of 12 Best Practices to Simplify Flutter App Development, Instead of using the print function for debugging purposes, it’s recommended to use the logger package or Flutter’s built-in logger. These tools provide more control over log messages, such as logging levels and filtering options. Here’s an example using the logger package:
import 'package:logger/logger.dart';
void main() {
var logger = Logger();
logger.d('Debug message');
logger.e('Error message');
logger.w('Warning message');
}
7. Avoiding Explicit Initialization of Variables as Null for Cleaner Code in Flutter
7th of 12 Best Practices to Simplify Flutter App Development, In Flutter app development, explicitly initializing variables as null can lead to unnecessary code clutter and potential pitfalls. In this guide, we will explore the best practice of avoiding explicit initialization of variables as null and how it can result in cleaner and more concise code. By relying on Dart’s null safety features and adopting proper variable initialization practices, you can improve code readability and reduce the risk of null reference errors.
late String myVariable;
String myVariable = ''; // or any other appropriate initial/default value
8. Maximizing Performance with the ‘const’ Keyword in Flutter
8th of 12 Best Practices to Simplify Flutter App Development, The const
keyword is used to create compile-time constants in Dart. By using const
, you can improve performance by reducing unnecessary object allocations. It’s recommended to use const
for widgets or values that won’t change during runtime. Here’s an example:
const PI = 3.14;
class MyWidget extends StatelessWidget {
@override
Widget build(BuildContext context) {
return const Text('Hello, World!');
}
}
9. Use Efficient State Management for Flutter App Development
9th of 12 Best Practices to Simplify Flutter App Development, State management is crucial for building complex Flutter applications. There are various state management solutions available like Provider, Riverpod, BLoC, MobX, etc. Choose a state management approach that suits your application’s needs and facilitates the organization and sharing of state between widgets. Here’s an example using the Provider package:
class MyWidget extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Consumer<MyModel>(
builder: (context, myModel, child) {
return Text(myModel.data);
},
);
}
}
10. Designing Beautiful and Consistent UI with Material Design Guidelines in Flutter
10th of 12 Best Practices to Simplify Flutter App Development, Flutter follows the Material Design guidelines, which provide design principles and UI components for building visually appealing and intuitive applications. By adhering to these guidelines, you ensure consistency across different platforms and improve the user experience. Use Flutter’s built-in Material widgets and follow the design patterns recommended by Material Design. Here’s an example using a Material button:
RaisedButton(
onPressed: () {
// Action on button press
},
child: Text('Submit'),
);
11. Ensuring Quality and Reliability with Automated Tests in Flutter
11th of 12 Best Practices to Simplify Flutter App Development, Writing automated tests is essential to ensure the quality and stability of your Flutter app. Flutter provides a robust testing framework that allows you to write unit tests and widget tests. Unit tests verify the functionality of individual functions or classes, while widget tests validate the behavior and rendering of widgets. Here’s an example of a widget test:
void main() {
testWidgets('Widget Test', (WidgetTester tester) async {
await tester.pumpWidget(MyWidget());
expect(find.text('Hello, World!'), findsOneWidget);
});
}
In this comprehensive guide, we have explored 12 best practices to simplify Flutter app development in 2023. By incorporating these practices into your workflow, you can optimize your code, enhance performance, and deliver high-quality applications to your users.
We started by emphasizing the importance of keeping the build function pure, utilizing operators efficiently to reduce code length, and using streams only when necessary. We also discussed the benefits of using raw strings, employing relative imports, and leveraging the SizedBox widget for layout efficiency.
Furthermore, we highlighted the advantages of logging instead of print statements, avoiding explicit initialization of variables as null, and utilizing the const keyword for compile-time constants. We also touched upon the significance of state management, adhering to Material Design guidelines, and writing automated tests to ensure the quality and stability of your app.
By following these best practices, you can simplify your Flutter app development process, improve code readability, and enhance the overall user experience. Stay updated with the latest advancements in Flutter and continuously refine your development approach to stay ahead in the ever-evolving landscape of mobile app development.
Incorporate these best practices into your development routine, experiment with new techniques, and continuously strive for improvement. Happy Flutter app development in 2023!
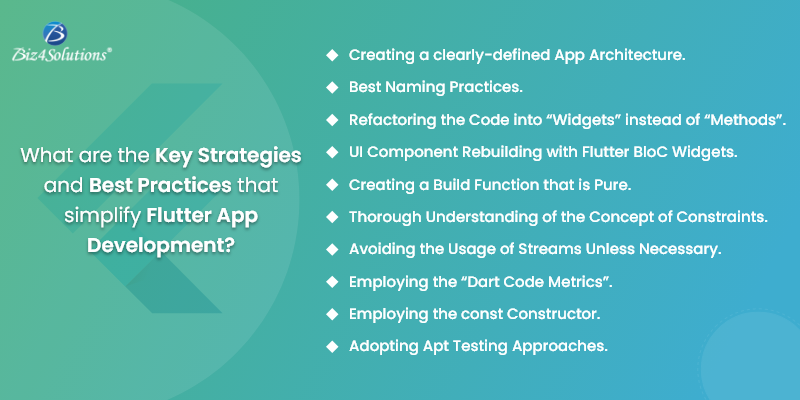
[…] Planning to build your app with Flutter then these 12 practices will simplify your flutter app development through the all process ranging from a code organization to UI design and testing. […]